As a data scientist and Python enthusiast, I understand the importance of writing efficient and optimized Python code. In this article, I’ll share my top techniques for optimizing Python code in various contexts, including data science, machine learning, web development, and data analysis.
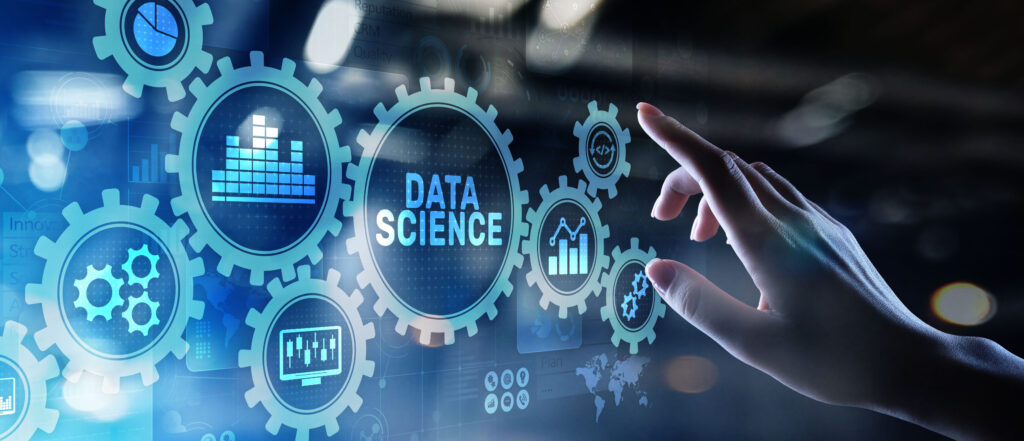
Python Code Optimization for Data Science
Data science projects often involve working with large datasets, which can slow down the performance of Python code. Here are some techniques you can use to optimize your code for data science applications:
- Use vectorized operations: Python has powerful libraries like NumPy and Pandas that allow you to perform vectorized operations on large datasets. These operations are optimized for speed and can significantly improve the performance of your code.
- Avoid using loops: Loops can be slow, especially when working with large datasets. Instead, try to use functions like apply(), map(), and filter() to perform operations on your data.
- Use generators: Generators are memory-efficient and can be faster than lists when iterating over large datasets.
- Profile your code: Use profiling tools like cProfile or PyCharm’s profiler to identify the bottlenecks in your code and optimize them.
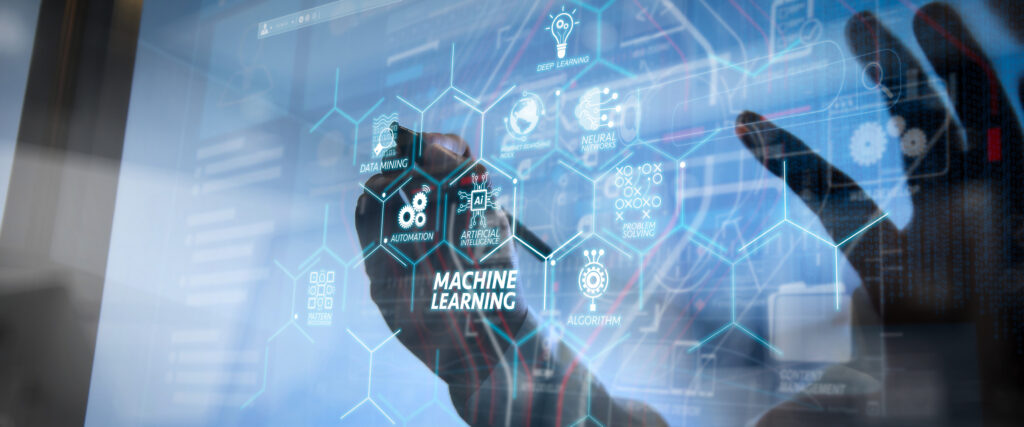
Techniques to Optimize Python Code for Machine Learning
Machine learning models can be resource-intensive, especially when working with large datasets. Here are some techniques you can use to optimize your Python code for machine learning applications:
- Use pre-trained models: Pre-trained models are models that have already been trained on large datasets and can be used for prediction tasks. This can save you time and resources, as you don’t have to train the model from scratch.
- Use sparse matrices: Sparse matrices are matrices that contain mostly zeros. They can be used to represent high-dimensional data and can save memory and computation time.
- Use GPU acceleration: GPUs are designed for parallel processing and can significantly speed up machine learning tasks. Libraries like TensorFlow and PyTorch have GPU support built-in.
- Use early stopping: Early stopping is a technique that stops the training process when the model stops improving. This can save time and resources by preventing the model from overfitting.
Python Code Optimization for Web Development
Web development projects often involve handling a large number of requests and processing data in real-time. Here are some techniques you can use to optimize your Python code for web development applications:
- Use caching: Caching can significantly speed up web applications by storing frequently accessed data in memory. Libraries like Redis and Memcached can be used for caching in Python.
- Use asynchronous programming: Asynchronous programming allows you to handle multiple requests concurrently, improving the responsiveness and performance of web applications. Libraries like asyncio and Trio can be used for asynchronous programming in Python.
- Use a lightweight web framework: Lightweight web frameworks like Flask and Bottle can be faster and more efficient than heavyweight frameworks like Django and Pyramid.
- Use a web server with a small footprint: Web servers like Gunicorn and uWSGI are lightweight and efficient, making them ideal for web applications.
How to Speed Up Python Code for Data Analysis
Data analysis projects can involve working with large datasets and performing complex operations. Here are some techniques you can use to speed up your Python code for data analysis:
- Use Cython: Cython is a language that is a superset of Python and allows you to write C extensions for Python. It can significantly speed up Python code for data analysis.
- Use Dask: Dask is a library that allows you to parallelize Python code for data analysis. It can scale to multiple cores and even multiple machines, making it ideal for large datasets.
- Use memory mapping: Memory mapping allows you to map a file to memory and access it like a large array. This can significantly speed up data analysis tasks.
In conclusion, Python code optimization is crucial for achieving optimal performance and productivity in various applications, including data science, machine learning, web development, and data analysis. By implementing these techniques, you can optimize your Python code for various applications and improve its performance and productivity.